y86-64 konekieli¶
Osaamistavoitteet: Käskykanta-arkkitehtuurit ja toteutus y86-suorittimessa.
Suorittimen hardistoteutus perustuu mikroarkkitehtuuriin, joka kuvaa ja toteuttaa suorittimen ohjelmoijalle näkyvän käskykanta-arkkitehtuurin (engl. Instruction set architecture, ISA), josta konekieli on yksi osa. Konekieli on tietokoneohjelman alimman tason laitteistoriippuvainen esitystapa suorittimen äidinkielellä. Konekielen käskyn muodostavat bitit peilautuvat suoraan fyysisten logiikka/mikropiirien sisäisiin portteihin, joiden sisältämän informaation perusteella suoritin toteuttaa käskyt sekvenssilogiikalla.
Edellisessä materiaalissa kävimme läpi y86-64 -assemblykielen käskyt ja tässä kappaleessa esitämme niitä vastaavan käskykanta-arkkitehtuurin ja konekielen toteutuksen.
Käskykanta-arkkitehtuuri¶
Käskykanta-arkkitehtuuri (ISA) siis kuvaa suorittimen toteutuksen niinkuin se ohjelmoijalle näkyy. ISA koostuu seuraavista osa-alueista:
- Tietotyypit mitkä suoritin ymmärtää: ml. 2-komplementtilukuesitys ja liukulukuesitys
- Rekisterit, eli suorittimen sisäinen muisti
- Muistin osoitusmuodot: suora, rekisteri, epäsuora
- Moderneissa suorittimissa erilaisia osoitusmuotoja voi olla jopa kymmeniä!
- Suorittimen tilabitit ja -rekisteri
- Sekvenssilogiikan (ja käyttöjärjestelmän) vaatimat ohjelman suorittamiseen liittyvät tilatiedot: erilaiset virhetilanteet, ym
- Konekielen käskykanta, jossa käskyt jaetaan seuraavasti
- Aritmeettiset operaatiot
- Loogiset operaatiot
- Siirto-operaatiot
- Ohjelman suoritusta ohjaavat käskyt
- Muistin arkkitehtuuri, virtuaalinen muistiavaruus: konekielisten ohjelmien näkemä muisti yhtenäisenä lohkona jossa jokainen muistipaikka koostuu sanoista.
- Muistiosoitteet alkavat (virtuaalisesti) ohjelmalle nollasta, riippumatta siitä missä lohko fyysisesti sijaitsee keskusmuistissa.
- Muistiosoitukset tehdään tällä virtuaalimuistin osoitteella
- Pino, eli ohjelman ajonaikainen tilapäismuisti
- Keskeytysten ja poikkeustilanteiden (oletus)käsittelijät
- Ulkoiset liitynnät: I/O-rekisterit ja -osoiteavaruus
Nyt tietysti sama käskykanta-arkkitehtuuri voidaan toteuttaa erilaisilla mikroarkkitehtuureilla. Esimerkkinä x86- (tai Intelin IA-32- ja Intel64-)käskykanta-arkkitehtuurit, joille on lähes identtisesti yhteensopivat toteutukset eri valmistajien suorittimissa: Intel, AMD, SPARC, jne. Lisäksi eri valmistajat ovat aikojen saatossa laajentaneet käskykantaa omilla suoritinkohtaisilla toteutuksillaan.
y86-konekielen käskyt¶
Konekielen käskyt koostuvat kahdesta osasta:
- Operaatiokoodi (engl. opcode), joka kertoo suoritettavan käskyn tyypin tai käskyryhmän ja sen alifunktion. Yksi tai useampi tavu.
- Operandit : vakioarvo / muisti / rekisteri eri osoitusmuodoissa. Yksi tai useampi tavu.
Assemblykielessä sama jako osiin nähdään selvästi, kun meillä on ihmisen ymmärtämä käskykoodi (=käskyn nimi) ja sille (tässä) maksimissaan kaksi operandia.
rrmovq %rax,%rbx
Operandit¶
Konekielessä voidaan käyttää erilaisia operandeja:
- Vakioarvoja, eli luvut noudattavat erityisesti konekielessä 2-komplementtiesitystä.
- Suoria muistiosoitteita, ovat vastaavasti lukuja.
- Rekisteriosoitusta varten jokaisella rekisterillä on oma numerokoodinsa.
y86-64:sessa rekisterikoodi on nelibittinen (2^4 = 16 rekisteriä), joten yhdellä tavulla kätevästi saadaan esitettyä kaikki suorittimen rekisterit. Tässä erotetaan lähderekisteri (engl. source) ja tulosrekisteri (kohderekisteri, engl. destination). Tässä Numero
0xF
tarkoittaa tyhjää operandia, eli rekisteri-operandia ei käskyssä tarvita, esimerkiksi kun operandi on vakioarvo tai muistista haettu arvo.Rekisteri | Numerokoodi | Rekisteri | Numerokoodi |
%rax | 0 | %r8 | 8 |
%rcx | 1 | %r9 | 9 |
%rdx | 2 | %r10 | A |
%rbx | 3 | %r11 | B |
%rsp | 4 | %r12 | C |
%rbp | 5 | %r13 | D |
%rsi | 6 | %r14 | E |
%rdi | 7 | - | F |
Muistiosoitusta varten muistiosoite esitetään vakioarvona, tässä siis 8-tavun (64-bittiä) mittaisena lukuna. (Ja muistetaan se erilainen tavujärjestys.) Käskykoodista riippuen vakioarvo osataan sitten tulkita näissä käskyissä muistiosoitteeksi.
Käskyjen koodaus ja rakenne¶
Näinollen jokaista y86-64 -konekielen käskyä (operaatiokoodi + operandit) vastaa 1-10 tavua seuraavasti.
Käskyn ensimmäinen tavu (1) kertoo käskyn operaatiokoodin, jonka ensimmäinen osa käskytyyppi ja toinen osa käskyn toiminto (funktio).
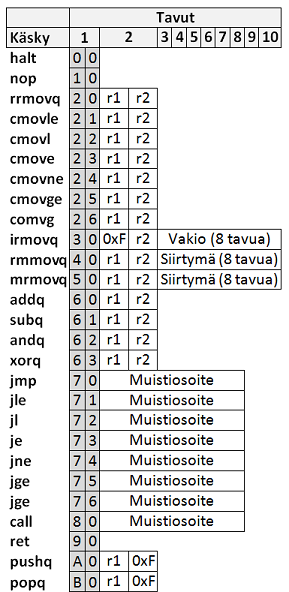
Hox! Samankaltaisten käskyjen hierarkinen ryhmittely käskykoodin perusteella.
Käskyn seuraavat tavut (2-10) kertovat käskyn operandit.
- Kahden rekisteri-operandin tapauksessa operandien kuvaamiseen riittää yksi tavu:
r1
jar2
. - Vakiota tai muistiosoitteita käytettäessä tarvitaan lisäksi 8 tavua muistipaikan, muistisiirtymän (engl. offset) tai arvon kuvaamiseen. Tässä tavujärjestys on little endian. Tällöin toinen rekisterioperandi, eli se mitä ei käskyssä tarvita, on merkitty koodilla
F
. - Siirtymä (engl. offset) tarkoittaa epäsuorassa muistiosoituksessa mahdollisesti olevaa numeroarvoa, jolla voidaan toteutta suhteellinen muistiosoitus, jossa siis haun muistiosoite on annettu muistiosoite plus/miinus siirtymän arvo.
Esimerkkejä y86-assemblykäskyistä konekielisenä esityksenä.
Assembly | Konekielen käsky | Selitys |
cmovg %rax,%rcx | 2601 | cmovg=26, rax=0, rcx=1 |
irmovq $0x1234,%rsi | 30f63412000000000000 | irmovq=30, F=ei-reg, rsi=6, vakioarvo=1234 |
addq %rdi,%rax | 6070 | addq=60, rdi=7, rax=0 |
jne 0x3c | 743c00000000000000 | jne=74, osoite=3c |
ret | 90 | ret=90 |
pushq %rbx | a03f | pushq=a0, rbx=3, F=ei-reg |
Mikäli konekielen käskyn operaatiokoodi tai operandit ovat virheellisiä, suoritin asettuu poikkeustilaan (tilalippu) ja aiheuttaa sovitun poikkeuksen
INS
. Jos muistiosoitteessa on virhe, aiheutuu siitä taas poikkeus ADR
. Nyt kuitenkaan varsinaista poikkeuksien käsittelijämekanismia y86-prosessorissa ei ole, vaan kaikki poikkeukset pysäyttävät sen toiminnan.
Lopuksi¶
Aikoinaan Suomessakin 80-luvun kotimikrobuumin aikana suosituimman Commodore 64-kotimikron suorittimen 6510:n käskykanta löytyy täältä (s. 8-9). Huomataan, että tässä oikeassa prosessorissa oli käytössä vain kolme rekisteriä A (akku), X ja Y! Lisäksi vielä aritmeettisissa operaatioissa rekisterien käyttöön oli jokaiselle rekisterille oma käsky. Myös muistista lukemiseen/kirjoittamiseen löytyy omat käskynsä. Käskykanta on siis vielä alkeellisempi verrattuna y86-prosessoriin.. ja datakirjakin vain 10 sivua vs modernin x86:sen noin 8000 sivua!!
Anna palautetta
Kommentteja materiaalista?